C# sample code to call the load calculation
web service for carton loads
This sample code calls the web service with passing the shipment
information including two box sizes and cargo list. It takes the load
plans from the web service back and shows the calculation to the
console. 3D graphics are saved at GIF images files at a client computer.
Picture 1> Result at the
Console
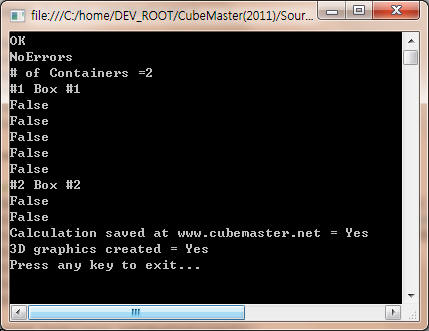
Picture 2> Result at a folder 'C:\Temp\Images'
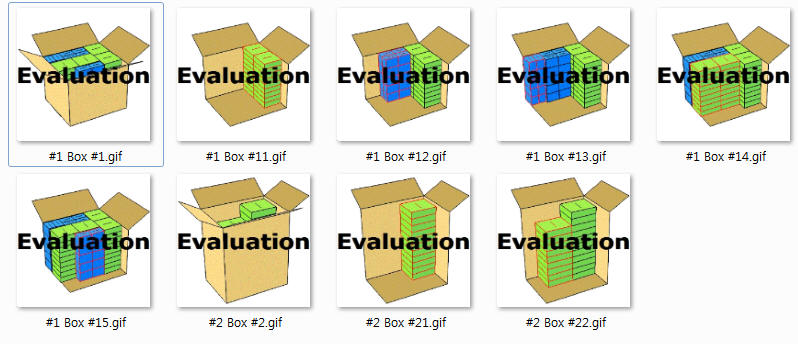
|
|
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
//for the shake to save JPG
using System.Drawing;
using System.Drawing.Imaging;
namespace ConsoleApplication1
{
class Program
{
static void
Main(string[] args)
{
//Create caculation
WebService.CalculationClient client = new WebService.CalculationClient();
//Create account
WebService.Account Account = new WebService.Account();
Account.UserID = "TRIAL1";//replace with your account
Account.Password = "0000";//replace with your account
Account.Company = "Trials";//replace with your account
//Create options
WebService.Options Options = new WebService.Options();
Options.CalculationSaved = true;
Options.GraphicsCreated = true;
Options.GraphicsImageWidth = 300;
Options.GraphicsImageDepth = 300;
Options.UOM = WebService.Options.UOMEnum.UnitMetric;//0=UnitEnglish, 1=UnitMetric, 2=UnitHighMetric
//Create shipment
WebService.Shipment Shipment = client.GetShipment();
Shipment.Title = "New Shipment Sample for Vehicle Load";
Shipment.Description = "in C# code";
//Create cargoes
WebService.Cargo NewCargo1 = new WebService.Cargo();
NewCargo1.Name = "First Cargo";
NewCargo1.Length = 500;
NewCargo1.Width = 500;
NewCargo1.Height = 500;
NewCargo1.Qty = 40;
Shipment.Cargoes.Add(NewCargo1);
WebService.Cargo NewCargo2 = new WebService.Cargo();
NewCargo2.Name = "Second Cargo";
NewCargo2.Length = 700;
NewCargo2.Width = 800;
NewCargo2.Height = 700;
NewCargo2.Qty = 56;
Shipment.Cargoes.Add(NewCargo2);
//Define cartons
WebService.Container NewContainer1 = new WebService.Container();
NewContainer1.ContainerType =
WebService.Container.ContainerTypeEnum.Carton;
NewContainer1.CartonType = WebService.Container.CartonTypeEnum.RSC;
NewContainer1.Name = "Box #1";
NewContainer1.Length = 200;
NewContainer1.Width= 230;
NewContainer1.Height= 180;
Shipment.Containers.Add(NewContainer1);
WebService.Container NewContainer2 = new WebService.Container();
NewContainer2.ContainerType =
WebService.Container.ContainerTypeEnum.Carton;
NewContainer2.CartonType = WebService.Container.CartonTypeEnum.RSC;
NewContainer2.Name = "Box #2";
NewContainer2.Length = 140;
NewContainer2.Width = 200;
NewContainer2.Height = 230;
//Define Rules
Shipment.Rules.IsWeightLimited = false;
//Run
WebService.LoadPlan LoadPlan = client.Run(Shipment, Account, Options);
//Show the return
Console.WriteLine(LoadPlan.Status);
if (LoadPlan.Status == "OK")
{
//Access the restuls
Console.WriteLine("# of Containers =" + LoadPlan.FilledContainers.Count);
try
{
foreach (WebService.FilledContainer Container in
LoadPlan.FilledContainers)
{
Console.WriteLine(Container.Name);
byte[] bytes = Convert.FromBase64String(Container.BytesImage3D);
System.IO.MemoryStream ms = new System.IO.MemoryStream(bytes);
Image img = Image.FromStream(ms);
img.Save("c:\\temp\\images\\" + Container.Name + ".gif",
System.Drawing.Imaging.ImageFormat.Gif);
//Spaces
foreach (WebService.SpaceInContainer Space in Container.Spaces)
{
Console.WriteLine(Space.IsEmpty);
if (Space.IsEmpty == false)
{
bytes = Convert.FromBase64String(Space.BytesImage3D);
ms = new System.IO.MemoryStream(bytes);
img = Image.FromStream(ms);
img.Save("c:\\temp\\images\\" + Container.Name + Space.Sequence +
".gif", System.Drawing.Imaging.ImageFormat.Gif);
}
}
//Manifest (Load List)
foreach (WebService.ManifestLine ManifestLine in Container.Manifest)
{
Console.WriteLine(ManifestLine.Sequence + ":" + ManifestLine.Cargo.Name
+ " x " + ManifestLine.CargoQty);
}
}
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
}//LoadPlan.Status == "OK"
Console.WriteLine("Calculation saved at www.cubemaster.net = " + (Options.CalculationSaved
== true ? "Yes" : "No"));
Console.WriteLine("3D graphics created = " + (Options.GraphicsCreated ==
true ? "Yes" : "No"));
Console.WriteLine("Press any key to exit...");
Console.ReadKey(true);
client.Close();
}
}
} |
|
|